Enhanced RFP and Proposal Writing Services: Streamlining Your Success
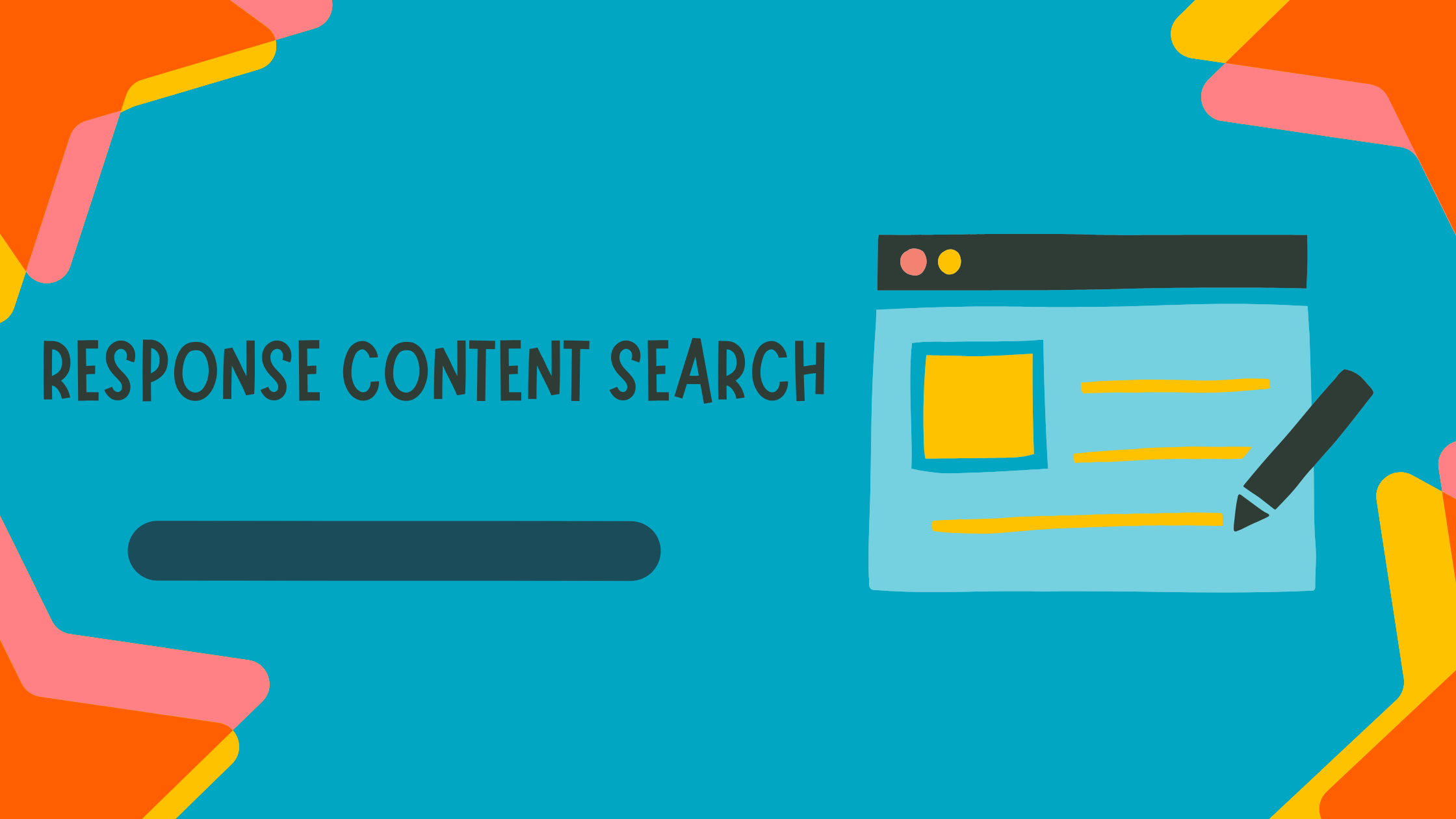
Need Help with Your Bid?
Get in touch by filling out the form and one of our advisors will be in contact.
Contact Us
In our ever-evolving digital world, understanding how to effectively retrieve and handle response content from HTTP requests is crucial for developers. The Python Requests library simplifies this process, allowing us to make web requests and access response data effortlessly. Knowing how to handle different types of responses, whether they are in JSON, text, or binary formats, is essential for efficient data processing.

When making HTTP requests, getting the content of a response can be done in various ways. For instance, we might use response.json()
for JSON data or response.content
for binary data. This flexibility enables us to handle a wide array of data types and structures, making our applications more robust and versatile.
Learning to properly work with response content not only enhances our programming skills but also saves time and reduces potential errors. Whether we're dealing with APIs, web scraping, or simple data retrieval, mastering these techniques will significantly improve our capabilities and efficiency.
Key Takeaways
- Understand different response content types with the Python Requests library.
- Properly handle response data to improve application robustness.
- Mastering these skills saves time and reduces errors in development.
Understanding HTTP Requests and Responses
Understanding HTTP requests and responses helps us grasp how data moves across the web. We'll explore the basics, the different methods used, and how to inspect the data we receive from servers.
Fundamentals of HTTP
HTTP, or Hypertext Transfer Protocol, is the foundation of communication on the web. When we visit a website, our browser sends an HTTP request to the server hosting that site. The server then responds with an HTTP response, which includes the requested data.
Requests and responses have both headers and bodies. Headers contain important metadata such as the URL, content type, and cookies. Meanwhile, the body holds the actual data sent or received. This process ensures smooth interaction between clients and servers.
Different HTTP Methods
HTTP methods define what kind of action we want to perform on the resource identified by the URL. The most common methods are:
- GET: Retrieves data from the server.
- POST: Sends data to the server to create a new resource.
- PUT: Updates an existing resource on the server.
- DELETE: Removes a resource from the server.
Each method serves a specific purpose. For example, when we fill out a form on a website and submit it, a POST request is sent to the server. Understanding these methods helps us tailor requests to achieve our desired outcomes efficiently.
Examining Response Objects
An HTTP response object contains the data sent back from the server after a request. Key components include:
- Status Code: Indicates the outcome of the request (e.g., 200 - OK, 404 - Not Found).
- Headers: Provide metadata about the response, like content type and length.
- Body: Contains the actual data, such as HTML, JSON, or images.
Examining these elements allows us to debug issues and understand the server's response. For instance, a status code of 200 means the request was successful, while a status code of 404 signals that the requested resource couldn't be found. By analyzing response objects, we can improve our web interactions and troubleshoot problems effectively.
Working with the Python Requests Library
We will explore how to install the Python Requests library, make basic HTTP requests, and utilize its advanced features.
Installation and Quickstart
To begin, we need to install the Requests library. It can be installed using pip, the package installer for Python:
pip install requests
Once installed, we can import it and start making requests. Let's make a quick GET request:
import requests
response = requests.get('https://api.github.com')
print(response.status_code)
In this example, we use the requests.get
method to send a GET request to the GitHub API. We then print the status code of the response.
Making Basic Requests
The Requests library makes it easy to perform common HTTP requests such as GET, POST, DELETE, and more. For example, a POST request can be made as follows:
response = requests.post('https://httpbin.org/post', data={'key': 'value'})
print(response.json())
This sends data to the specified URL. Similarly, we can handle responses in JSON format using response.json()
.
For deleting resources, we use the DELETE method:
response = requests.delete('https://httpbin.org/delete')
print(response.status_code)
We also explore sending and receiving data with GET requests and reading the response body using response.text
or response.content
.
Advanced Usage and Features
For more control, we can use the Session object to persist certain parameters across requests:
session = requests.Session()
session.headers.update({'Authorization': 'Bearer token'})
response = session.get('https://api.github.com/user/repos')
print(response.json())
We can handle non-blocking I/O by leveraging advanced features like streaming:
response = requests.get('https://api.github.com/events', stream=True)
for line in response.iter_lines():
if line:
print(line)
The Requests library also supports custom adapters for advanced networking needs or debugging by accessing the PreparedRequest object:
from requests.adapters import HTTPAdapter
session = requests.Session()
session.mount('http://', HTTPAdapter(max_retries=3))
response = session.get('http://httpbin.org/get')
print(response.status_code)
By using these tools, we can significantly enhance how we manage HTTP requests in our Python applications.
Data Handling in HTTP Communication

In HTTP communication, managing data is crucial to ensure that requests and responses are accurate and efficient. We must handle different types of data such as JSON, binary data, and textual data with the proper encodings.
Sending and Receiving Data
When we send data through an HTTP request, it can be in various formats like JSON, form data, or even raw bytes. The data, known as the payload, is included in the message body. To specify the type of data, we use headers like Content-Type
. For example, JSON data is typically sent with Content-Type: application/json
.
Receiving data is just as critical. When an HTTP response arrives, we need to correctly interpret this data. The response content might be HTML, XML, JSON, or even binary data. We use attributes like response.content
to get the raw response content and response.text
for text data.
Handling JSON and Binary Data
JSON is a common data format in web communication. When an HTTP response returns JSON data, we can easily parse it using response.json()
. This method allows us to access the data as a dictionary. It simplifies the handling of structured data such as API responses.
Binary data, like images or files, requires a different approach. We access binary response content using response.content
. For large files, streaming is more efficient. By setting stream=True
, we can download large files in chunks using response.iter_content()
. This helps us manage memory more effectively during the download.
Encoding and Character Sets
Handling text data correctly involves understanding character encoding. Typically, HTTP responses include a Content-Type
header with the character encoding specified, such as charset=UTF-8
. When we receive text data, we need to decode it using the correct character encoding to avoid issues like garbled text.
If a response lacks explicit encoding, we can use response.apparent_encoding
to guess the correct encoding. Ensuring proper encoding is vital for accurately displaying and processing text data. It guarantees that all characters, including special symbols, are correctly interpreted.
In summary, understanding how to handle different types of data in HTTP communication is essential for robust and efficient web interactions. Using the appropriate methods for sending, receiving, and decoding data ensures that applications work smoothly across various data formats and encodings.
Authentication and Error Handling
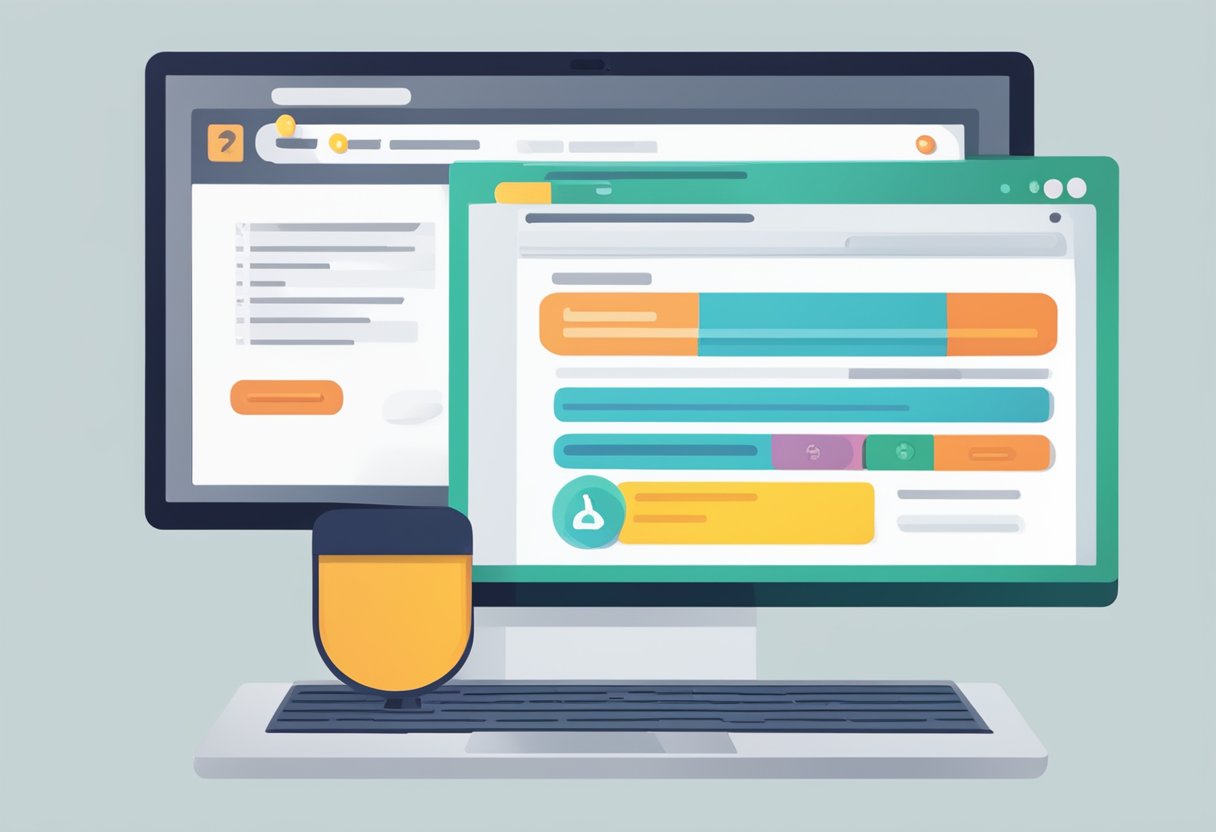
The key to a secure and robust application lies in proper authentication, session management, and error handling. We need to ensure smooth user interactions while maintaining tight security.
Implementing Authentication
Authentication is the process of verifying who a user is. In most systems, users provide a username and password. Using tools like Spring Security, we can set up custom authentication handlers. These handlers allow us to tailor the response when login attempts fail.
For example, a custom authentication handler can be used to log failed attempts or show custom error messages. When implementing SSL certificates, we secure the transmission of credentials over the internet, adding an extra layer of security.
Managing Sessions and Cookies
Sessions and cookies help us maintain the state of authenticated users. A session is created when a user logs in and is identified by a unique session ID. This ID is usually stored in a cookie on the user's browser.
Managing sessions involves ensuring they are valid and secure, as they can be targets for hijacking. Cookies can store session IDs and some user preferences, but they must be protected using the SameSite
attribute and set as HttpOnly
to prevent access by JavaScript.
Handling Errors and Exceptions
Handling errors effectively means providing useful feedback to users and keeping the system secure. We use HTTP response status codes to indicate the result of a request. For example, a 404
indicates a page not found, while a 500
indicates a server error.
In APIs, it is good practice to handle errors centrally using a global error handler. This helps in logging errors consistently and displaying user-friendly messages. For instance, in a REST API, we can use Spring to implement a Global Exception Handler, which catches exceptions and returns a structured error response.
In HTTP requests, using methods like raise_for_status()
in libraries such as Axios or the Python Requests library can help ensure we handle errors at the right place, allowing us to log the error and alert users accordingly.
Frequently Asked Questions
We often get questions about handling response content in Python, especially when dealing with web requests. Let's go over the most common queries and best practices.
How can you retrieve response content using Python?
We can retrieve response content using the requests
library in Python. By sending an HTTP request with requests.get(url)
, we receive a response object. This object allows us to access the retrieved content through its .content
or .text
attributes.
What methods are available for searching within a response content in Python?
To search within response content, we can use Python's string methods or regular expressions. Methods like .find()
or .contains()
help us locate specific terms. For more complex searches, regular expressions are more powerful, allowing pattern matching within the content.
How do you convert a server's response content to a JSON object in Python?
We can convert response content to a JSON object using the .json()
method of the response object. Once we have a response, we call response.json()
, which parses the JSON content and returns a Python dictionary.
What distinguishes response.text from response.content in a Python requests library?
The difference between .text
and .content
lies in encoding. The .text
attribute returns the content decoded as a string using the response's apparent encoding. In contrast, the response.content returns the raw bytes of the content.
What are the best practices for handling raw responses in web requests?
First, we should always check the response status code using response.status_code
to ensure the request was successful. Using exception handling to catch errors is also crucial. Finally, if handling large responses, processing the content in chunks can improve efficiency.
In Python, how can you handle different content types within a HTTP response?
Depending on the content type, we use different methods. For HTML or text, we use .text
. For JSON, .json()
is appropriate. For other binary data like images or files, response.content is used. Always check response.headers['Content-Type']
to know the content type and handle it accordingly.
Ready to start your search?
Get in touch by filling out the form to the right and one of our advisors will curate a personalised selection for you.
Get in touchBlogs. Guides. Helpful advice.

Mastering Proposal and RFP Writing for Government and Public Sector Opportunities

Proposal and RFP Writing Services: Enhancing Public Sector Tender Outcomes
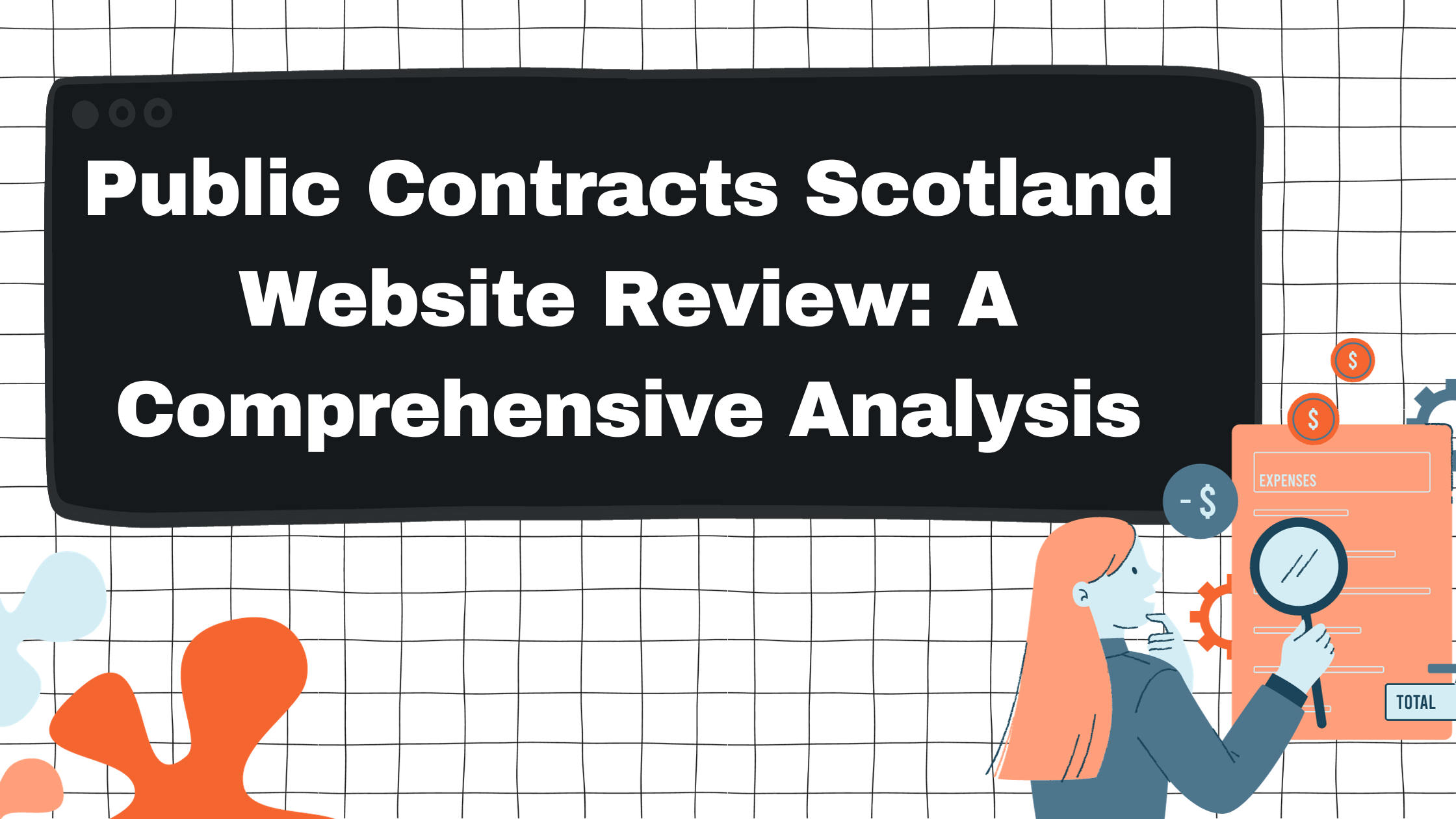